OAuth 2.0 资源服务器 JWT
JWT 的最小依赖项
大多数资源服务器支持都包含在 spring-security-oauth2-resource-server
中。但是,解码和验证 JWT 的支持位于 spring-security-oauth2-jose
中,这意味着两者都需要才能使支持 JWT 编码的 Bearer 令牌的资源服务器正常工作。
JWT 的最小配置
当使用 Spring Boot 时,将应用程序配置为资源服务器包括两个基本步骤。首先,包含所需的依赖项,其次,指示授权服务器的位置。
指定授权服务器
在 Spring Boot 应用程序中,要指定使用哪个授权服务器,只需执行以下操作
spring:
security:
oauth2:
resourceserver:
jwt:
issuer-uri: https://idp.example.com/issuer
其中 idp.example.com/issuer
是授权服务器将发出的 JWT 令牌的 iss
声明中包含的值。资源服务器将使用此属性进行进一步的自动配置,发现授权服务器的公钥,并随后验证传入的 JWT。
要使用 issuer-uri 属性,还必须为真,即 idp.example.com/issuer/.well-known/openid-configuration 、idp.example.com/.well-known/openid-configuration/issuer 或 idp.example.com/.well-known/oauth-authorization-server/issuer 是授权服务器支持的端点。此端点称为 提供程序配置 端点或 授权服务器元数据 端点。 |
就是这样!
启动预期
当使用此属性和这些依赖项时,资源服务器将自动配置自身以验证 JWT 编码的 Bearer 令牌。
它通过确定性的启动过程实现这一点
-
查询提供程序配置或授权服务器元数据端点以获取
jwks_url
属性 -
查询
jwks_url
端点以获取支持的算法 -
配置验证策略以查询
jwks_url
以获取找到的算法的有效公钥 -
配置验证策略以针对
idp.example.com
验证每个 JWT 的iss
声明。
此过程的结果是,授权服务器必须启动并接收请求才能使资源服务器成功启动。
如果资源服务器查询授权服务器时(在适当的超时时间内)授权服务器已关闭,则启动将失败。 |
运行时预期
应用程序启动后,资源服务器将尝试处理任何包含 Authorization: Bearer
标头的请求
GET / HTTP/1.1
Authorization: Bearer some-token-value # Resource Server will process this
只要指示了此方案,资源服务器就会尝试根据 Bearer 令牌规范处理请求。
给定一个格式良好的 JWT,资源服务器将
-
针对启动期间从
jwks_url
端点获取并与 JWT 匹配的公钥验证其签名 -
验证 JWT 的
exp
和nbf
时间戳以及 JWT 的iss
声明,以及 -
将每个范围映射到以
SCOPE_
为前缀的权限。
随着授权服务器提供新的密钥,Spring Security 将自动轮换用于验证 JWT 的密钥。 |
默认情况下,生成的 Authentication#getPrincipal
是 Spring Security Jwt
对象,而 Authentication#getName
映射到 JWT 的 sub
属性(如果存在)。
从这里开始,请考虑跳转到
JWT 身份验证的工作原理
接下来,让我们看看 Spring Security 用于在基于 servlet 的应用程序(例如我们刚刚看到的应用程序)中支持 JWT 身份验证的架构组件。
JwtAuthenticationProvider
是一个 AuthenticationProvider
实现,它利用 JwtDecoder
和 JwtAuthenticationConverter
来验证 JWT。
让我们看看 JwtAuthenticationProvider
如何在 Spring Security 中工作。该图详细说明了来自 读取 Bearer 令牌 的图中 AuthenticationManager
如何工作。
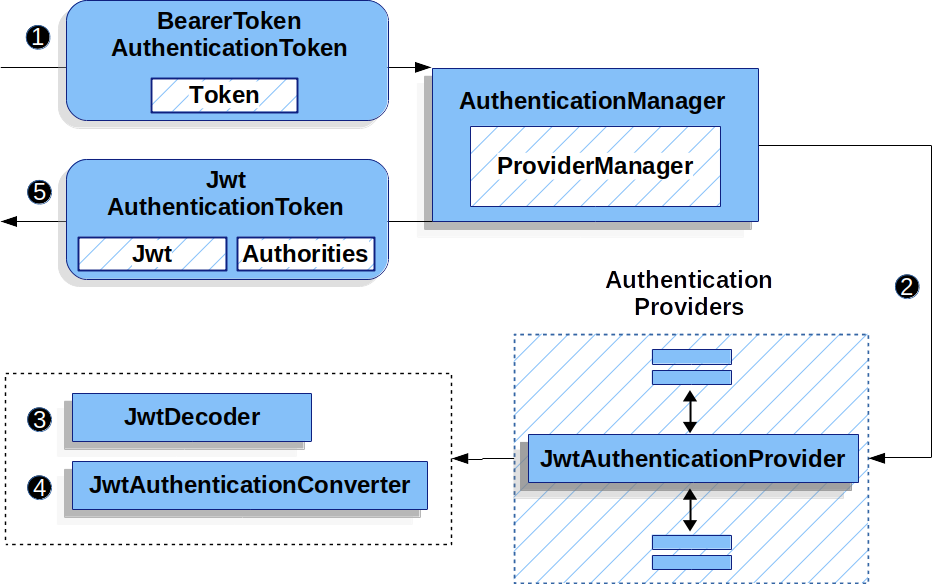
JwtAuthenticationProvider
用法 来自 读取 Bearer 令牌 的身份验证
Filter
将 BearerTokenAuthenticationToken
传递给由 ProviderManager
实现的 AuthenticationManager
。
ProviderManager
配置为使用类型为 JwtAuthenticationProvider
的 AuthenticationProvider。
JwtAuthenticationProvider
使用 JwtDecoder
解码、验证和校验 Jwt
。
然后,
JwtAuthenticationProvider
使用 JwtAuthenticationConverter
将 Jwt
转换为授予的权限的 Collection
。
当身份验证成功时,返回的
Authentication
的类型为 JwtAuthenticationToken
,并且其主体是配置的 JwtDecoder
返回的 Jwt
。最终,返回的 JwtAuthenticationToken
将由身份验证 Filter
设置在 SecurityContextHolder
上。
直接指定授权服务器 JWK 集 URI
如果授权服务器不支持任何配置端点,或者如果资源服务器必须能够独立于授权服务器启动,则也可以提供 jwk-set-uri
spring:
security:
oauth2:
resourceserver:
jwt:
issuer-uri: https://idp.example.com
jwk-set-uri: https://idp.example.com/.well-known/jwks.json
JWK 集 URI 没有标准化,但通常可以在授权服务器的文档中找到。 |
因此,资源服务器在启动时不会 ping 授权服务器。我们仍然指定 issuer-uri
,以便资源服务器仍然验证传入 JWT 上的 iss
声明。
此属性也可以直接在 DSL 上提供。 |
提供受众
如已所见,issuer-uri
属性验证 iss
声明;这是发送 JWT 的方。
Boot 还具有 audiences
属性来验证 aud
声明;这是 JWT 发送到的方。
资源服务器的受众可以这样指示
spring:
security:
oauth2:
resourceserver:
jwt:
issuer-uri: https://idp.example.com
audiences: https://my-resource-server.example.com
如果需要,您还可以 以编程方式添加 aud 验证。 |
结果将是,如果 JWT 的 iss
声明不是 idp.example.com
,并且其 aud
声明不包含其列表中的 my-resource-server.example.com
,则验证将失败。
覆盖或替换 Boot 自动配置
Spring Boot 代表资源服务器生成两个 @Bean
。
第一个是 SecurityFilterChain
,它将应用程序配置为资源服务器。包含 spring-security-oauth2-jose
时,此 SecurityFilterChain
如下所示
-
Java
-
Kotlin
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2ResourceServer((oauth2) -> oauth2.jwt(Customizer.withDefaults()));
return http.build();
}
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
jwt { }
}
}
return http.build()
}
如果应用程序不公开 SecurityFilterChain
bean,则 Spring Boot 将公开上述默认 bean。
替换它就像在应用程序中公开 bean 一样简单
-
Java
-
Kotlin
import static org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope;
@Configuration
@EnableWebSecurity
public class MyCustomSecurityConfiguration {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.requestMatchers("/messages/**").access(hasScope("message:read"))
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2
.jwt(jwt -> jwt
.jwtAuthenticationConverter(myConverter())
)
);
return http.build();
}
}
import org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope
@Configuration
@EnableWebSecurity
class MyCustomSecurityConfiguration {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize("/messages/**", hasScope("message:read"))
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
jwt {
jwtAuthenticationConverter = myConverter()
}
}
}
return http.build()
}
}
以上要求任何以 /messages/
开头的 URL 具有 message:read
的范围。
oauth2ResourceServer
DSL 上的方法也将覆盖或替换自动配置。
例如,Spring Boot 创建的第二个 @Bean
是 JwtDecoder
,它 将 String
令牌解码为经过验证的 Jwt
实例
-
Java
-
Kotlin
@Bean
public JwtDecoder jwtDecoder() {
return JwtDecoders.fromIssuerLocation(issuerUri);
}
@Bean
fun jwtDecoder(): JwtDecoder {
return JwtDecoders.fromIssuerLocation(issuerUri)
}
调用 JwtDecoders#fromIssuerLocation 将调用提供程序配置或授权服务器元数据端点以派生 JWK 集 URI。 |
如果应用程序不公开 JwtDecoder
bean,则 Spring Boot 将公开上述默认 bean。
并且可以使用 jwkSetUri()
覆盖其配置或使用 decoder()
替换。
或者,如果您根本不使用 Spring Boot,则可以在 XML 中指定这两个组件(过滤器链和 JwtDecoder
)。
过滤器链指定如下
-
Xml
<http>
<intercept-uri pattern="/**" access="authenticated"/>
<oauth2-resource-server>
<jwt decoder-ref="jwtDecoder"/>
</oauth2-resource-server>
</http>
以及 JwtDecoder
如下所示
-
Xml
<bean id="jwtDecoder"
class="org.springframework.security.oauth2.jwt.JwtDecoders"
factory-method="fromIssuerLocation">
<constructor-arg value="${spring.security.oauth2.resourceserver.jwt.jwk-set-uri}"/>
</bean>
使用 jwkSetUri()
授权服务器的 JWK 集 URI 可以 作为配置属性配置,也可以在 DSL 中提供。
-
Java
-
Kotlin
-
Xml
@Configuration
@EnableWebSecurity
public class DirectlyConfiguredJwkSetUri {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2
.jwt(jwt -> jwt
.jwkSetUri("https://idp.example.com/.well-known/jwks.json")
)
);
return http.build();
}
}
@Configuration
@EnableWebSecurity
class DirectlyConfiguredJwkSetUri {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
jwt {
jwkSetUri = "https://idp.example.com/.well-known/jwks.json"
}
}
}
return http.build()
}
}
<http>
<intercept-uri pattern="/**" access="authenticated"/>
<oauth2-resource-server>
<jwt jwk-set-uri="https://idp.example.com/.well-known/jwks.json"/>
</oauth2-resource-server>
</http>
使用 jwkSetUri()
优先于任何配置属性。
使用 decoder()
比 jwkSetUri()
更强大的是 decoder()
,它将完全替换 JwtDecoder
的任何 Boot 自动配置。
-
Java
-
Kotlin
-
Xml
@Configuration
@EnableWebSecurity
public class DirectlyConfiguredJwtDecoder {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2
.jwt(jwt -> jwt
.decoder(myCustomDecoder())
)
);
return http.build();
}
}
@Configuration
@EnableWebSecurity
class DirectlyConfiguredJwtDecoder {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
jwt {
jwtDecoder = myCustomDecoder()
}
}
}
return http.build()
}
}
<http>
<intercept-uri pattern="/**" access="authenticated"/>
<oauth2-resource-server>
<jwt decoder-ref="myCustomDecoder"/>
</oauth2-resource-server>
</http>
公开 JwtDecoder
@Bean
或者,公开 JwtDecoder
@Bean
与 decoder()
具有相同的效果。您可以使用 jwkSetUri
构造一个,如下所示
-
Java
-
Kotlin
@Bean
public JwtDecoder jwtDecoder() {
return NimbusJwtDecoder.withJwkSetUri(jwkSetUri).build();
}
@Bean
fun jwtDecoder(): JwtDecoder {
return NimbusJwtDecoder.withJwkSetUri(jwkSetUri).build()
}
或者您可以使用颁发者并让 NimbusJwtDecoder
在调用 build()
时查找 jwkSetUri
,如下所示
-
Java
-
Kotlin
@Bean
public JwtDecoder jwtDecoder() {
return NimbusJwtDecoder.withIssuerLocation(issuer).build();
}
@Bean
fun jwtDecoder(): JwtDecoder {
return NimbusJwtDecoder.withIssuerLocation(issuer).build()
}
或者,如果默认值对您有效,您也可以使用 JwtDecoders
,它除了配置解码器的验证器之外,还执行上述操作。
-
Java
-
Kotlin
@Bean
public JwtDecoders jwtDecoder() {
return JwtDecoders.fromIssuerLocation(issuer);
}
@Bean
fun jwtDecoder(): JwtDecoders {
return JwtDecoders.fromIssuerLocation(issuer)
}
配置受信任的算法
默认情况下,NimbusJwtDecoder
以及资源服务器,仅信任并验证使用 RS256
的令牌。
您可以通过 Spring Boot、NimbusJwtDecoder 生成器 或 JWK 集响应 来自定义此功能。
通过 Spring Boot
设置算法的最简单方法是作为属性
spring:
security:
oauth2:
resourceserver:
jwt:
jws-algorithms: RS512
jwk-set-uri: https://idp.example.org/.well-known/jwks.json
使用生成器
但是,为了获得更大的功能,我们可以使用 NimbusJwtDecoder
附带的生成器
-
Java
-
Kotlin
@Bean
JwtDecoder jwtDecoder() {
return NimbusJwtDecoder.withIssuerLocation(this.issuer)
.jwsAlgorithm(RS512).build();
}
@Bean
fun jwtDecoder(): JwtDecoder {
return NimbusJwtDecoder.withIssuerLocation(this.issuer)
.jwsAlgorithm(RS512).build()
}
多次调用 jwsAlgorithm
将配置 NimbusJwtDecoder
以信任多个算法,如下所示
-
Java
-
Kotlin
@Bean
JwtDecoder jwtDecoder() {
return NimbusJwtDecoder.withIssuerLocation(this.issuer)
.jwsAlgorithm(RS512).jwsAlgorithm(ES512).build();
}
@Bean
fun jwtDecoder(): JwtDecoder {
return NimbusJwtDecoder.withIssuerLocation(this.issuer)
.jwsAlgorithm(RS512).jwsAlgorithm(ES512).build()
}
或者,您可以调用 jwsAlgorithms
-
Java
-
Kotlin
@Bean
JwtDecoder jwtDecoder() {
return NimbusJwtDecoder.withIssuerLocation(this.issuer)
.jwsAlgorithms(algorithms -> {
algorithms.add(RS512);
algorithms.add(ES512);
}).build();
}
@Bean
fun jwtDecoder(): JwtDecoder {
return NimbusJwtDecoder.withIssuerLocation(this.issuer)
.jwsAlgorithms {
it.add(RS512)
it.add(ES512)
}.build()
}
来自 JWK 集响应
由于 Spring Security 的 JWT 支持基于 Nimbus,因此您也可以使用其所有强大的功能。
例如,Nimbus 具有一个 JWSKeySelector
实现,它将根据 JWK 集 URI 响应选择算法集。您可以使用它来生成 NimbusJwtDecoder
,如下所示
-
Java
-
Kotlin
@Bean
public JwtDecoder jwtDecoder() {
// makes a request to the JWK Set endpoint
JWSKeySelector<SecurityContext> jwsKeySelector =
JWSAlgorithmFamilyJWSKeySelector.fromJWKSetURL(this.jwkSetUrl);
DefaultJWTProcessor<SecurityContext> jwtProcessor =
new DefaultJWTProcessor<>();
jwtProcessor.setJWSKeySelector(jwsKeySelector);
return new NimbusJwtDecoder(jwtProcessor);
}
@Bean
fun jwtDecoder(): JwtDecoder {
// makes a request to the JWK Set endpoint
val jwsKeySelector: JWSKeySelector<SecurityContext> = JWSAlgorithmFamilyJWSKeySelector.fromJWKSetURL<SecurityContext>(this.jwkSetUrl)
val jwtProcessor: DefaultJWTProcessor<SecurityContext> = DefaultJWTProcessor()
jwtProcessor.jwsKeySelector = jwsKeySelector
return NimbusJwtDecoder(jwtProcessor)
}
信任单个非对称密钥
比使用 JWK 集端点支持资源服务器更简单的方法是硬编码 RSA 公钥。可以通过 Spring Boot 或 使用生成器 提供公钥。
通过 Spring Boot
通过 Spring Boot 指定密钥非常简单。密钥的位置可以这样指定
spring:
security:
oauth2:
resourceserver:
jwt:
public-key-location: classpath:my-key.pub
或者,为了允许更复杂的查找,您可以后处理 RsaKeyConversionServicePostProcessor
-
Java
-
Kotlin
@Bean
BeanFactoryPostProcessor conversionServiceCustomizer() {
return beanFactory ->
beanFactory.getBean(RsaKeyConversionServicePostProcessor.class)
.setResourceLoader(new CustomResourceLoader());
}
@Bean
fun conversionServiceCustomizer(): BeanFactoryPostProcessor {
return BeanFactoryPostProcessor { beanFactory ->
beanFactory.getBean<RsaKeyConversionServicePostProcessor>()
.setResourceLoader(CustomResourceLoader())
}
}
指定密钥的位置
key.location: hfds://my-key.pub
然后自动连接值
-
Java
-
Kotlin
@Value("${key.location}")
RSAPublicKey key;
@Value("\${key.location}")
val key: RSAPublicKey? = null
信任单个对称密钥
使用单个对称密钥也很简单。您可以简单地加载 SecretKey
并使用相应的 NimbusJwtDecoder
生成器,如下所示
-
Java
-
Kotlin
@Bean
public JwtDecoder jwtDecoder() {
return NimbusJwtDecoder.withSecretKey(this.key).build();
}
@Bean
fun jwtDecoder(): JwtDecoder {
return NimbusJwtDecoder.withSecretKey(key).build()
}
配置授权
从 OAuth 2.0 授权服务器发出的 JWT 通常具有 scope
或 scp
属性,指示它已被授予的范围(或权限),例如
{ …, "scope" : "messages contacts"}
在这种情况下,资源服务器将尝试将这些范围强制转换为授予的权限列表,并在每个范围前添加字符串 "SCOPE_"。
这意味着要使用从 JWT 派生的范围保护端点或方法,相应的表达式应包含此前缀。
-
Java
-
Kotlin
-
Xml
import static org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope;
@Configuration
@EnableWebSecurity
public class DirectlyConfiguredJwkSetUri {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.requestMatchers("/contacts/**").access(hasScope("contacts"))
.requestMatchers("/messages/**").access(hasScope("messages"))
.anyRequest().authenticated()
)
.oauth2ResourceServer(OAuth2ResourceServerConfigurer::jwt);
return http.build();
}
}
import org.springframework.security.oauth2.core.authorization.OAuth2AuthorizationManagers.hasScope;
@Configuration
@EnableWebSecurity
class DirectlyConfiguredJwkSetUri {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize("/contacts/**", hasScope("contacts"))
authorize("/messages/**", hasScope("messages"))
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
jwt { }
}
}
return http.build()
}
}
<http>
<intercept-uri pattern="/contacts/**" access="hasAuthority('SCOPE_contacts')"/>
<intercept-uri pattern="/messages/**" access="hasAuthority('SCOPE_messages')"/>
<oauth2-resource-server>
<jwt jwk-set-uri="https://idp.example.org/.well-known/jwks.json"/>
</oauth2-resource-server>
</http>
或者类似的方法安全性
-
Java
-
Kotlin
@PreAuthorize("hasAuthority('SCOPE_messages')")
public List<Message> getMessages(...) {}
@PreAuthorize("hasAuthority('SCOPE_messages')")
fun getMessages(): List<Message> { }
手动提取权限
但是,在许多情况下,此默认设置是不够的。例如,某些授权服务器不使用scope
属性,而是使用自己的自定义属性。或者,在其他时候,资源服务器可能需要将属性或属性组合适配为内部化的权限。
为此,Spring Security 附带了JwtAuthenticationConverter
,它负责将Jwt
转换为Authentication
。默认情况下,Spring Security 会将JwtAuthenticationProvider
与JwtAuthenticationConverter
的默认实例连接起来。
作为配置JwtAuthenticationConverter
的一部分,您可以提供一个辅助转换器,用于将Jwt
转换为授予权限的Collection
。
假设您的授权服务器在名为authorities
的自定义声明中传递权限。在这种情况下,您可以配置JwtAuthenticationConverter
应检查的声明,如下所示
-
Java
-
Kotlin
-
Xml
@Bean
public JwtAuthenticationConverter jwtAuthenticationConverter() {
JwtGrantedAuthoritiesConverter grantedAuthoritiesConverter = new JwtGrantedAuthoritiesConverter();
grantedAuthoritiesConverter.setAuthoritiesClaimName("authorities");
JwtAuthenticationConverter jwtAuthenticationConverter = new JwtAuthenticationConverter();
jwtAuthenticationConverter.setJwtGrantedAuthoritiesConverter(grantedAuthoritiesConverter);
return jwtAuthenticationConverter;
}
@Bean
fun jwtAuthenticationConverter(): JwtAuthenticationConverter {
val grantedAuthoritiesConverter = JwtGrantedAuthoritiesConverter()
grantedAuthoritiesConverter.setAuthoritiesClaimName("authorities")
val jwtAuthenticationConverter = JwtAuthenticationConverter()
jwtAuthenticationConverter.setJwtGrantedAuthoritiesConverter(grantedAuthoritiesConverter)
return jwtAuthenticationConverter
}
<http>
<intercept-uri pattern="/contacts/**" access="hasAuthority('SCOPE_contacts')"/>
<intercept-uri pattern="/messages/**" access="hasAuthority('SCOPE_messages')"/>
<oauth2-resource-server>
<jwt jwk-set-uri="https://idp.example.org/.well-known/jwks.json"
jwt-authentication-converter-ref="jwtAuthenticationConverter"/>
</oauth2-resource-server>
</http>
<bean id="jwtAuthenticationConverter"
class="org.springframework.security.oauth2.server.resource.authentication.JwtAuthenticationConverter">
<property name="jwtGrantedAuthoritiesConverter" ref="jwtGrantedAuthoritiesConverter"/>
</bean>
<bean id="jwtGrantedAuthoritiesConverter"
class="org.springframework.security.oauth2.server.resource.authentication.JwtGrantedAuthoritiesConverter">
<property name="authoritiesClaimName" value="authorities"/>
</bean>
您还可以配置权限前缀为不同的值。而不是在每个权限前添加SCOPE_
,您可以将其更改为ROLE_
,如下所示
-
Java
-
Kotlin
-
Xml
@Bean
public JwtAuthenticationConverter jwtAuthenticationConverter() {
JwtGrantedAuthoritiesConverter grantedAuthoritiesConverter = new JwtGrantedAuthoritiesConverter();
grantedAuthoritiesConverter.setAuthorityPrefix("ROLE_");
JwtAuthenticationConverter jwtAuthenticationConverter = new JwtAuthenticationConverter();
jwtAuthenticationConverter.setJwtGrantedAuthoritiesConverter(grantedAuthoritiesConverter);
return jwtAuthenticationConverter;
}
@Bean
fun jwtAuthenticationConverter(): JwtAuthenticationConverter {
val grantedAuthoritiesConverter = JwtGrantedAuthoritiesConverter()
grantedAuthoritiesConverter.setAuthorityPrefix("ROLE_")
val jwtAuthenticationConverter = JwtAuthenticationConverter()
jwtAuthenticationConverter.setJwtGrantedAuthoritiesConverter(grantedAuthoritiesConverter)
return jwtAuthenticationConverter
}
<http>
<intercept-uri pattern="/contacts/**" access="hasAuthority('SCOPE_contacts')"/>
<intercept-uri pattern="/messages/**" access="hasAuthority('SCOPE_messages')"/>
<oauth2-resource-server>
<jwt jwk-set-uri="https://idp.example.org/.well-known/jwks.json"
jwt-authentication-converter-ref="jwtAuthenticationConverter"/>
</oauth2-resource-server>
</http>
<bean id="jwtAuthenticationConverter"
class="org.springframework.security.oauth2.server.resource.authentication.JwtAuthenticationConverter">
<property name="jwtGrantedAuthoritiesConverter" ref="jwtGrantedAuthoritiesConverter"/>
</bean>
<bean id="jwtGrantedAuthoritiesConverter"
class="org.springframework.security.oauth2.server.resource.authentication.JwtGrantedAuthoritiesConverter">
<property name="authorityPrefix" value="ROLE_"/>
</bean>
或者,您可以通过调用JwtGrantedAuthoritiesConverter#setAuthorityPrefix("")
完全删除前缀。
为了获得更大的灵活性,DSL 支持使用任何实现Converter<Jwt, AbstractAuthenticationToken>
的类完全替换转换器。
-
Java
-
Kotlin
static class CustomAuthenticationConverter implements Converter<Jwt, AbstractAuthenticationToken> {
public AbstractAuthenticationToken convert(Jwt jwt) {
return new CustomAuthenticationToken(jwt);
}
}
// ...
@Configuration
@EnableWebSecurity
public class CustomAuthenticationConverterConfig {
@Bean
public SecurityFilterChain filterChain(HttpSecurity http) throws Exception {
http
.authorizeHttpRequests(authorize -> authorize
.anyRequest().authenticated()
)
.oauth2ResourceServer(oauth2 -> oauth2
.jwt(jwt -> jwt
.jwtAuthenticationConverter(new CustomAuthenticationConverter())
)
);
return http.build();
}
}
internal class CustomAuthenticationConverter : Converter<Jwt, AbstractAuthenticationToken> {
override fun convert(jwt: Jwt): AbstractAuthenticationToken {
return CustomAuthenticationToken(jwt)
}
}
// ...
@Configuration
@EnableWebSecurity
class CustomAuthenticationConverterConfig {
@Bean
open fun filterChain(http: HttpSecurity): SecurityFilterChain {
http {
authorizeRequests {
authorize(anyRequest, authenticated)
}
oauth2ResourceServer {
jwt {
jwtAuthenticationConverter = CustomAuthenticationConverter()
}
}
}
return http.build()
}
}
配置验证
使用最少的 Spring Boot 配置,指示授权服务器的发行者 URI,资源服务器将默认验证iss
声明以及exp
和nbf
时间戳声明。
在需要自定义验证的情况下,资源服务器附带了两个标准验证器,并且还接受自定义的OAuth2TokenValidator
实例。
自定义时间戳验证
JWT 通常具有有效期窗口,窗口的开始时间由nbf
声明指示,结束时间由exp
声明指示。
但是,每个服务器都可能遇到时钟漂移,这可能导致令牌对一台服务器而言已过期,但对另一台服务器而言却没有过期。随着分布式系统中协作服务器数量的增加,这可能会导致一些实现上的问题。
资源服务器使用JwtTimestampValidator
来验证令牌的有效期窗口,并且可以使用clockSkew
来缓解上述问题。
-
Java
-
Kotlin
@Bean
JwtDecoder jwtDecoder() {
NimbusJwtDecoder jwtDecoder = (NimbusJwtDecoder)
JwtDecoders.fromIssuerLocation(issuerUri);
OAuth2TokenValidator<Jwt> withClockSkew = new DelegatingOAuth2TokenValidator<>(
new JwtTimestampValidator(Duration.ofSeconds(60)),
new JwtIssuerValidator(issuerUri));
jwtDecoder.setJwtValidator(withClockSkew);
return jwtDecoder;
}
@Bean
fun jwtDecoder(): JwtDecoder {
val jwtDecoder: NimbusJwtDecoder = JwtDecoders.fromIssuerLocation(issuerUri) as NimbusJwtDecoder
val withClockSkew: OAuth2TokenValidator<Jwt> = DelegatingOAuth2TokenValidator(
JwtTimestampValidator(Duration.ofSeconds(60)),
JwtIssuerValidator(issuerUri))
jwtDecoder.setJwtValidator(withClockSkew)
return jwtDecoder
}
默认情况下,资源服务器配置了 60 秒的时钟偏差。 |
配置自定义验证器
使用OAuth2TokenValidator
API 检查aud
声明非常简单。
-
Java
-
Kotlin
OAuth2TokenValidator<Jwt> audienceValidator() {
return new JwtClaimValidator<List<String>>(AUD, aud -> aud.contains("messaging"));
}
fun audienceValidator(): OAuth2TokenValidator<Jwt?> {
return JwtClaimValidator<List<String>>(AUD) { aud -> aud.contains("messaging") }
}
或者,为了获得更多控制,您可以实现自己的OAuth2TokenValidator
。
-
Java
-
Kotlin
static class AudienceValidator implements OAuth2TokenValidator<Jwt> {
OAuth2Error error = new OAuth2Error("custom_code", "Custom error message", null);
@Override
public OAuth2TokenValidatorResult validate(Jwt jwt) {
if (jwt.getAudience().contains("messaging")) {
return OAuth2TokenValidatorResult.success();
} else {
return OAuth2TokenValidatorResult.failure(error);
}
}
}
// ...
OAuth2TokenValidator<Jwt> audienceValidator() {
return new AudienceValidator();
}
internal class AudienceValidator : OAuth2TokenValidator<Jwt> {
var error: OAuth2Error = OAuth2Error("custom_code", "Custom error message", null)
override fun validate(jwt: Jwt): OAuth2TokenValidatorResult {
return if (jwt.audience.contains("messaging")) {
OAuth2TokenValidatorResult.success()
} else {
OAuth2TokenValidatorResult.failure(error)
}
}
}
// ...
fun audienceValidator(): OAuth2TokenValidator<Jwt> {
return AudienceValidator()
}
然后,要将其添加到资源服务器中,只需指定JwtDecoder
实例即可。
-
Java
-
Kotlin
@Bean
JwtDecoder jwtDecoder() {
NimbusJwtDecoder jwtDecoder = (NimbusJwtDecoder)
JwtDecoders.fromIssuerLocation(issuerUri);
OAuth2TokenValidator<Jwt> audienceValidator = audienceValidator();
OAuth2TokenValidator<Jwt> withIssuer = JwtValidators.createDefaultWithIssuer(issuerUri);
OAuth2TokenValidator<Jwt> withAudience = new DelegatingOAuth2TokenValidator<>(withIssuer, audienceValidator);
jwtDecoder.setJwtValidator(withAudience);
return jwtDecoder;
}
@Bean
fun jwtDecoder(): JwtDecoder {
val jwtDecoder: NimbusJwtDecoder = JwtDecoders.fromIssuerLocation(issuerUri) as NimbusJwtDecoder
val audienceValidator = audienceValidator()
val withIssuer: OAuth2TokenValidator<Jwt> = JwtValidators.createDefaultWithIssuer(issuerUri)
val withAudience: OAuth2TokenValidator<Jwt> = DelegatingOAuth2TokenValidator(withIssuer, audienceValidator)
jwtDecoder.setJwtValidator(withAudience)
return jwtDecoder
}
如前所述,您也可以在 Boot 中配置aud 验证。 |
配置声明集映射
Spring Security 使用Nimbus库来解析 JWT 并验证其签名。因此,Spring Security 受制于 Nimbus 对每个字段值的解释以及如何将其强制转换为 Java 类型。
例如,由于 Nimbus 保持与 Java 7 兼容,因此它不使用Instant
来表示时间戳字段。
并且完全有可能使用不同的库或用于 JWT 处理,这可能会做出自己的强制转换决策,需要进行调整。
或者,简单地说,资源服务器可能希望出于特定于域的原因向 JWT 添加或删除声明。
出于这些目的,资源服务器支持使用MappedJwtClaimSetConverter
映射 JWT 声明集。
自定义单个声明的转换
默认情况下,MappedJwtClaimSetConverter
将尝试将声明强制转换为以下类型。
声明 |
Java 类型 |
|
|
|
|
|
|
|
|
|
|
|
|
|
|
可以使用MappedJwtClaimSetConverter.withDefaults
配置单个声明的转换策略。
-
Java
-
Kotlin
@Bean
JwtDecoder jwtDecoder() {
NimbusJwtDecoder jwtDecoder = NimbusJwtDecoder.withIssuerLocation(issuer).build();
MappedJwtClaimSetConverter converter = MappedJwtClaimSetConverter
.withDefaults(Collections.singletonMap("sub", this::lookupUserIdBySub));
jwtDecoder.setClaimSetConverter(converter);
return jwtDecoder;
}
@Bean
fun jwtDecoder(): JwtDecoder {
val jwtDecoder = NimbusJwtDecoder.withIssuerLocation(issuer).build()
val converter = MappedJwtClaimSetConverter
.withDefaults(mapOf("sub" to this::lookupUserIdBySub))
jwtDecoder.setClaimSetConverter(converter)
return jwtDecoder
}
这将保留所有默认值,但会覆盖sub
的默认声明转换器。
添加声明
MappedJwtClaimSetConverter
也可用于添加自定义声明,例如,以适应现有系统。
-
Java
-
Kotlin
MappedJwtClaimSetConverter.withDefaults(Collections.singletonMap("custom", custom -> "value"));
MappedJwtClaimSetConverter.withDefaults(mapOf("custom" to Converter<Any, String> { "value" }))
删除声明
并且删除声明也很简单,使用相同的 API。
-
Java
-
Kotlin
MappedJwtClaimSetConverter.withDefaults(Collections.singletonMap("legacyclaim", legacy -> null));
MappedJwtClaimSetConverter.withDefaults(mapOf("legacyclaim" to Converter<Any, Any> { null }))
重命名声明
在更复杂的场景中,例如同时查询多个声明或重命名声明,资源服务器接受任何实现Converter<Map<String, Object>, Map<String,Object>>
的类。
-
Java
-
Kotlin
public class UsernameSubClaimAdapter implements Converter<Map<String, Object>, Map<String, Object>> {
private final MappedJwtClaimSetConverter delegate =
MappedJwtClaimSetConverter.withDefaults(Collections.emptyMap());
public Map<String, Object> convert(Map<String, Object> claims) {
Map<String, Object> convertedClaims = this.delegate.convert(claims);
String username = (String) convertedClaims.get("user_name");
convertedClaims.put("sub", username);
return convertedClaims;
}
}
class UsernameSubClaimAdapter : Converter<Map<String, Any?>, Map<String, Any?>> {
private val delegate = MappedJwtClaimSetConverter.withDefaults(Collections.emptyMap())
override fun convert(claims: Map<String, Any?>): Map<String, Any?> {
val convertedClaims = delegate.convert(claims)
val username = convertedClaims["user_name"] as String
convertedClaims["sub"] = username
return convertedClaims
}
}
然后,可以像往常一样提供该实例。
-
Java
-
Kotlin
@Bean
JwtDecoder jwtDecoder() {
NimbusJwtDecoder jwtDecoder = NimbusJwtDecoder.withIssuerLocation(issuer).build();
jwtDecoder.setClaimSetConverter(new UsernameSubClaimAdapter());
return jwtDecoder;
}
@Bean
fun jwtDecoder(): JwtDecoder {
val jwtDecoder: NimbusJwtDecoder = NimbusJwtDecoder.withIssuerLocation(issuer).build()
jwtDecoder.setClaimSetConverter(UsernameSubClaimAdapter())
return jwtDecoder
}
配置超时
默认情况下,资源服务器使用 30 秒的连接和套接字超时来协调与授权服务器的通信。
在某些情况下,这可能太短了。此外,它没有考虑更复杂的模式,例如回退和发现。
要调整资源服务器连接到授权服务器的方式,NimbusJwtDecoder
接受RestOperations
的实例。
-
Java
-
Kotlin
@Bean
public JwtDecoder jwtDecoder(RestTemplateBuilder builder) {
RestOperations rest = builder
.setConnectTimeout(Duration.ofSeconds(60))
.setReadTimeout(Duration.ofSeconds(60))
.build();
NimbusJwtDecoder jwtDecoder = NimbusJwtDecoder.withIssuerLocation(issuer).restOperations(rest).build();
return jwtDecoder;
}
@Bean
fun jwtDecoder(builder: RestTemplateBuilder): JwtDecoder {
val rest: RestOperations = builder
.setConnectTimeout(Duration.ofSeconds(60))
.setReadTimeout(Duration.ofSeconds(60))
.build()
return NimbusJwtDecoder.withIssuerLocation(issuer).restOperations(rest).build()
}
此外,默认情况下,资源服务器会在内存中缓存授权服务器的 JWK 集 5 分钟,您可能需要调整它。此外,它没有考虑更复杂的缓存模式,例如逐出或使用共享缓存。
要调整资源服务器缓存 JWK 集的方式,NimbusJwtDecoder
接受Cache
的实例。
-
Java
-
Kotlin
@Bean
public JwtDecoder jwtDecoder(CacheManager cacheManager) {
return NimbusJwtDecoder.withIssuerLocation(issuer)
.cache(cacheManager.getCache("jwks"))
.build();
}
@Bean
fun jwtDecoder(cacheManager: CacheManager): JwtDecoder {
return NimbusJwtDecoder.withIssuerLocation(issuer)
.cache(cacheManager.getCache("jwks"))
.build()
}
给定Cache
时,资源服务器将使用 JWK 集 URI 作为键,并将 JWK 集 JSON 作为值。
Spring 不是缓存提供程序,因此您需要确保包含相应的依赖项,例如spring-boot-starter-cache 和您最喜欢的缓存提供程序。 |
无论是套接字还是缓存超时,您可能希望直接使用 Nimbus。为此,请记住NimbusJwtDecoder 附带一个构造函数,该构造函数采用 Nimbus 的JWTProcessor 。 |